오늘 git으로 협업하기 위한 강의를 들었는데 이해가 되지 않는 부분이 있어서
다시 보면서 머릿속에서 정리를 한 다음에 TIL로 써야 할 것 같다!
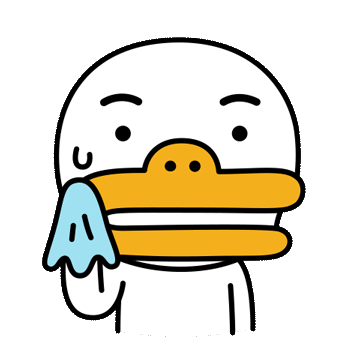
달리기 경주
Java 풀이 코드
public static String[] solution(String[] players, String[] callings) {
// for문 2개를 이용해서 선수를 찾으면 프로그래머스 테스크 케이스 8번부터
// 시간 초과가 떠서 HashMap을 사용!
HashMap<String, Integer> humanHashMap = new HashMap<String, Integer>();
for(int i = 0 ; i < players.length ; i ++){
humanHashMap.put(players[i],i); // key,value 쌍으로 선수 이름에 value부여
}
for(String called: callings) {
int index = humanHashMap.get(called); // 불려지는 선수의 value를 index에 대입
String forwardHuman = players[index-1]; // 앞질러질 선수를 구해놓음
humanHashMap.put(called,index - 1); // 해시맵의 key, value값을 바꿔줌
humanHashMap.put(forwardHuman,index); // 해시맵의 key, value값을 바꿔줌
players[index-1] = called; // 불려진 선수를 기존의 본인 앞 선수 순위로
players[index] = forwardHuman; // 앞질러진 선수는 불려진 선수 순위로
}
return players;
}
Python 풀이 코드
def solution(players, callings):
human_dict = {}
for i, j in enumerate(players):
human_dict[j] = i
for human in callings:
index = human_dict.get(human)
forward_human = players[index-1]
human_dict[human], human_dict[forward_human] = index - 1, index
players[index-1], players[index] = human, forward_human
return players
로직은 java와 python이 똑같다!
다만 java → HashMap / python → dictionary 자료형을 이용했다는 점!
추억점수
Java 풀이 코드
public static int[] solution(String[] name, int[] yearning, String[][] photo) {
int[] answer = new int[photo.length];
int a = 0;
HashMap<String,Integer> personHashMap = new HashMap<String,Integer>();
for(int i = 0 ; i < name.length ; i ++){
personHashMap.put(name[i],yearning[i]);
}
for(String[] photoPersonArray: photo){
int photoSum = 0;
for(String photoPerson : photoPersonArray){
if(personHashMap.get(photoPerson) != null) { // == if(personHashMap.containsKey(photoPerson)){
photoSum += personHashMap.get(photoPerson);
}
}
answer[a] += photoSum;
a ++;
}
return answer;
}
13번째 줄에 get으로 photoPerson을 찾았을 때 없으면 null값 반환
Python 풀이 코드
def solution(name, yearning, photo):
answer = []
person_dict = {}
for i in range(len(name)):
person_dict[name[i]] = yearning[i]
for i in photo:
photo_sum = 0
for j in i:
if j in name:
photo_sum += person_dict[j]
answer.append(photo_sum)
return answer
달리기 경주 문제처럼 HashMap과 dictionary 형을 이용하여 풀었고 비슷한 것 같다
이 문제도 java와 python 로직은 똑같고
이번엔 각 이름에 이름에 해당하는 점수를 부여하였다는 점이 달리기 경주 문제랑 차이가 난다고 볼 수 있다
python에서는 in을 이용해서 해당 이름이 있는지 없는지 확인하였지만
java에서는 HashMap.containsKey() 를 이용해서 확인하였다는 점이 달랐다
파이썬에 익숙해지기 위해서 프로그래머스 Lv.0 문제를 다 풀어보았고 내배캠을 시작하고부터 java로도 같이 풀기 시작해서 처음부터 java, python 같이 풀었더라면 java에도 많이 익숙해질 수 있었을 것 같은데 아쉽다는 생각이 들지만
Lv.1 문제들로 익숙해져 봐야겠다!
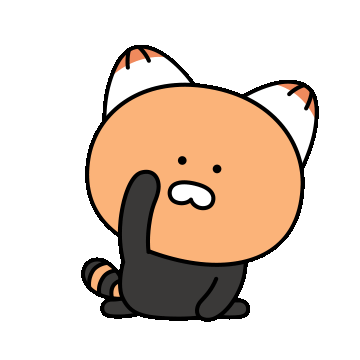
'TIL' 카테고리의 다른 글
2023-10-26 TIL(프로그래머스) (0) | 2023.10.26 |
---|---|
2023-10-25 TIL(객체 지향 프로그래밍의 특징, 프로그래머스) (0) | 2023.10.25 |
2023-10-23 TIL(컬랙션) (0) | 2023.10.23 |
2023-10-20 TIL (추상클래스와 인터페이스의 차이점) (2) | 2023.10.20 |
2023-10-19 TIL (git, 프로그래머스) (0) | 2023.10.19 |
댓글